String Variables
For our last variable in this guide, we’ll be dealing with a type of variable that can have a word as a value also known as a string. This variable is usually used when you want a certain string of letters to have meaning, and/or you’re not dealing with any math. Same as the other variables we’ve covered, we need to declare and initialize. To declare a string variable, state the variable, and then give it a name. It’s written like this, ‘String sVari;’. To initialize that string, write ‘sVari = “Hello”;’.
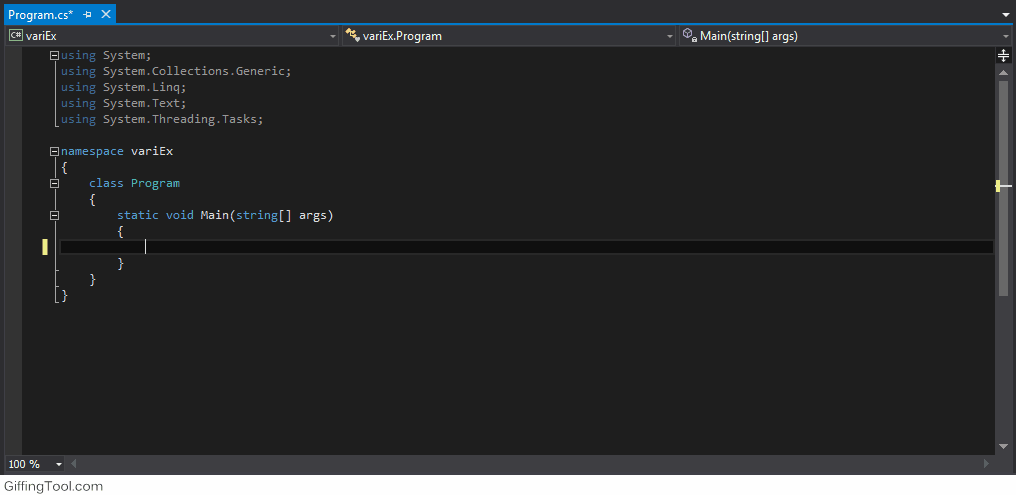
To display this variable properly, I will demonstrate a simple concatenating program to use this string variable. What you need to do is, create a new space, and add a second string by the name of 'sVari2' and give it a value of "World!". Then create a new line and add this code, 'Console.WriteLine(String.Concat(sVari, " ", sVari2));'. You will learn what this is in a future section. Also add the code 'Console.ReadLine();' to the end of the program.
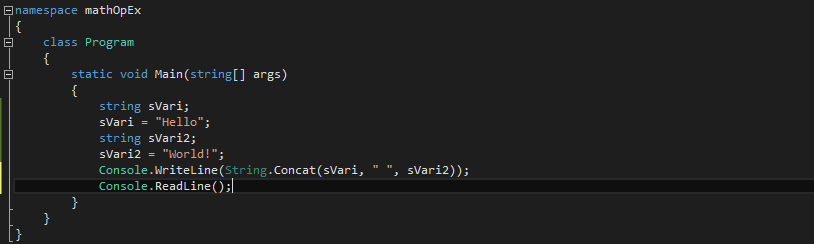
You will notice when you start the program, the two strings, "Hello" and "World!" have been put together to form a phrase.
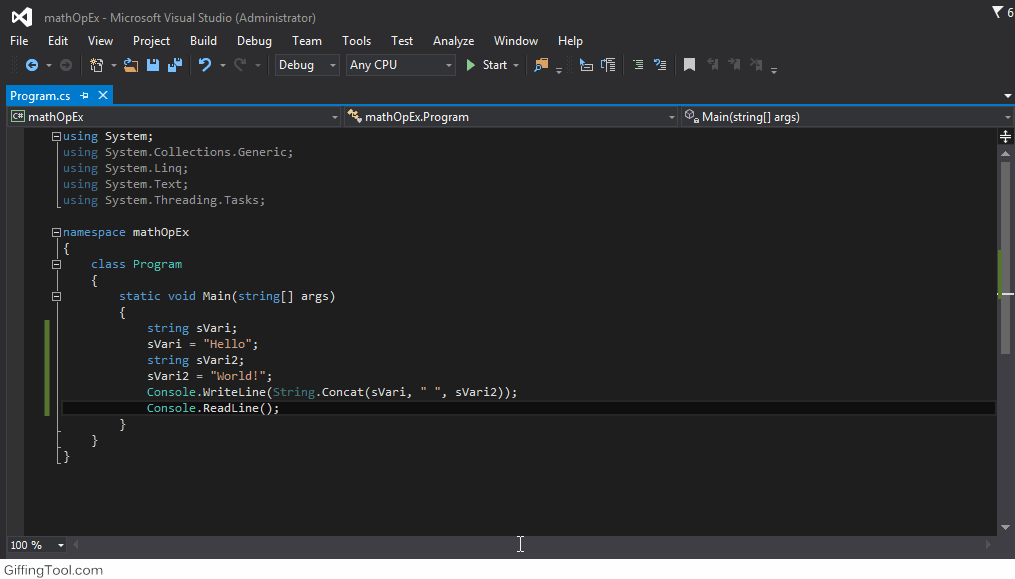
Note – You can combine the declaring and the initializing stage in the same sequence or on the same line. To do that, you need to take the variable name, put it beside the name your giving it, and then you can initialize its value. This works for all the variables I have showed you, for example ‘String strVar = “red”; ’.
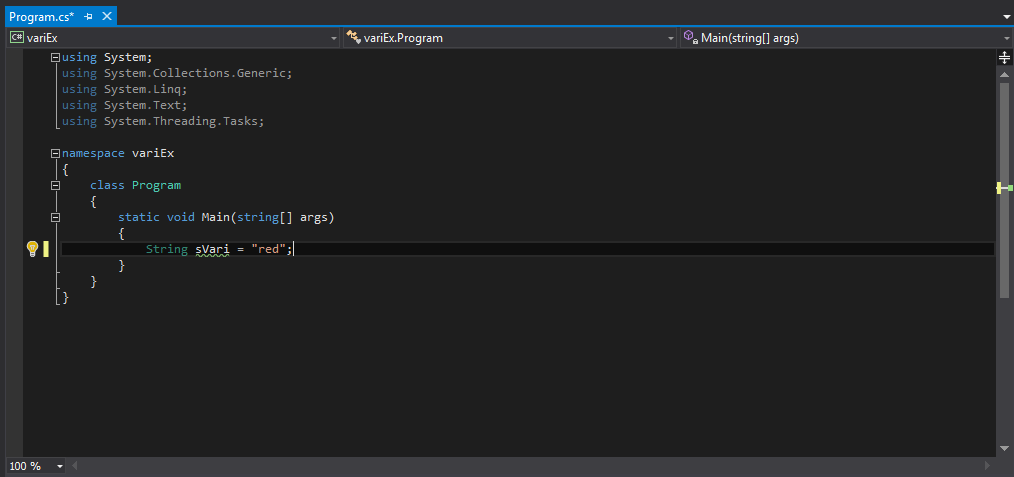
With that, concludes this section on variables you can use to represent things and make them communicate with each other in C#. These are probably one of the most important things in this guide because these skills can carry over to most other programming languages logistically. The next section for this guide will be covering how to get input out of your user, with both strings and numbers.